Keywords: CAN message, VB6.0, libTSCAN
Table of Contents for this article
01、About Demo Project
Brief description:
VB6.0 programming language calls libTSCAN interface to realize hardware scanning, connection, CAN message single frame sending, cycle sending, message receiving, message filtering.
Typical application requirements.
For the VB project project which is still under maintenance and development, it is necessary to call TSCAN hardware to realize the message sending and receiving and other operations.
02. Technical background
03、Demo project realization
1. Add library files
To realize the operation of TOSUN series CAN/CANFD, LIN devices, it needs to be based on libTSCAN.dll, libTSH.dll, binlog.dll and liblog.dll dynamic link library files. The dependencies between them are shown in Figure 1 below.
The locations of libTSCAN.dll, libTSH.dll, binlog.dll and liblog.dll dynamic link library files can be obtained from the TSMaster installation directory. c:\Program Files (x86)\TOSUN\TSMaster\Data\SDK\examples\ Python\Linux\lib\libTOSUN\windows\x86
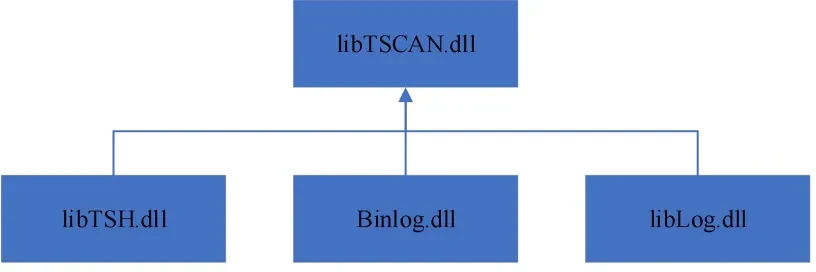
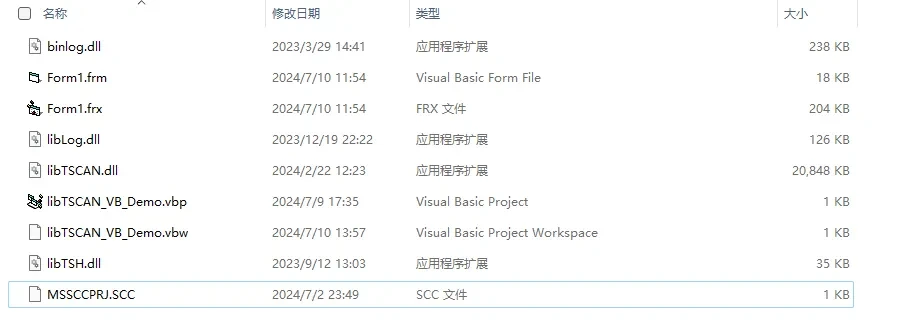
2. Declare the functions in libTSCAN.dll.
Use the Declare statement to declare the functions in libTSCAN.dll.
The syntax is as follows:
Private Declare [Function|Sub] External Function Name Lib "Library Filename" [Alias "Alias"] ([Argument List])
private: Optional, indicating that the declaration is visible within the current module. If you need to use it in multiple modules, you can use Public.
Declare: Keyword for declaring external functions.
FunctionmaybeSub: The choice is based on whether the external function returns a value (Function) or does not return a value (Sub).
External function name: the name of the function you use in your VB6.0 code.
Lib: Specifies the name of the DLL library file containing the function definition.
Library filename: the name of the DLL library file, excluding the extension (.lib or .dll).
Alias: Optional, if the function name in the DLL conflicts with the name in VB6.0 or does not conform to the naming rules of VB6.0, an alias can be used.
parameter list: The arguments to the function, including the name, type, and delivery method (ByVal or ByRef) of the argument.
'Load libTSCAN library, must be executed before calling other APIs'
Private Declare Sub initialize_lib_tscan_verbose Lib "libTSCAN.dll" (ByVal AEnableFIFO As Boolean, ByVal AEnableErrorFrame As Boolean, ByVal AUseHWTime As Boolean, ByVal ATSType As Long)
'Releases the libTSCAN library, which must be called before exiting the program after completing its use.
Private Declare Sub finalize_lib_tscan Lib "libTSCAN.dll" ()
'Query the device's manufacturer, product name, serial number, how many CAN channels it contains, whether it supports FDCAN, how many LIN channels, Flexray channels, and Ethernet channels'
Private Declare Function tscan_get_device_info_detail Lib "libTSCAN.dll" (ByVal ADeviceIndex As Long, ByRef AManufacturer As Long, ByRef AProduct As Long, ByRef ASerial As Long, ByRef ADeviceType As Long, ByRef ADeviceName As Long, ByRef ACANChannelCount As Long, ByRef AIsCANFD As Boolean, ByRef ALINChannelCount As Long, ByRef AFRChannelCount As Long, ByRef AEthernetChannelCount As Long) As Long
'Connected devices
Private Declare Function tscan_connect Lib "libTSCAN.dll" (ByVal ADeviceSerial As String, ByRef AHandle As Long) As Long
'Disconnect device
Private Declare Function tscan_disconnect_by_handle Lib "libTSCAN.dll" (ByVal AHandle As Long) As Long
'Query the number of TSCAN devices currently online
Private Declare Function tscan_scan_devices Lib "libTSCAN.dll" (ByRef ADeviceCount As Long) As Long
'Configure common CAN hardware channels
Private Declare Function tscan_config_can_by_baudrate Lib "libTSCAN.dll" (ByVal AHandle As Long, ByVal AChnIdx As Long, ByVal A120OhmConnected As Long, ByVal ARateKbps As Double, ByVal A120OhmConnected As Long) As Long ByVal ARateKbps As Double, ByVal A120OhmConnected As Long) As Long
'Configure FDCAN hardware channels; AControllerType[0: Normal CAN 1: ISO-FD 2: NoISO-FD] AControllerMode[0:Normal 1: ACKOff 2: Retricted 3: Internal Loop 4. External Loop]
Private Declare Function tscan_config_canfd_by_baudrate Lib "libTSCAN.dll" (ByVal AHandle As Long, ByVal AChnIdx As Long, ByVal AArbRateKbps As Double, ByVal ADataRateKbps As Double, ByVal AControllerType As Long, ByVal AControllerMode As Long, ByVal A120OhmConnected As Long) As Long
'Send CAN/CANFD message'
Private Declare Function tscan_transmit_can_sync Lib "libTSCAN.dll" (ByVal ADeviceHandle As Long, ByRef ACAN As TLIBCAN , ByVal ATimeoutMS As Long) As Long
Private Declare Function tscan_transmit_can_async Lib "libTSCAN.dll" (ByVal ADeviceHandle As Long, ByRef ACAN As ByVal ADeviceHandle As Long, ByRef ACAN As TLIBCAN) As Long
'Cycle sends CAN/CANFD message
'Add CAN cycle send message
Private Declare Function tscan_add_cyclic_msg_can Lib "libTSCAN.dll" (ByVal ADeviceHandle As Long, ByRef ACAN As ByVal ADeviceHandle As Long, ByRef ACAN As TLIBCAN, ByVal APeriodMS As Single) As Long
'Delete CAN Cycle Transmit message
Private Declare Function tscan_delete_cyclic_msg_can Lib "libTSCAN.dll" (ByVal ADeviceHandle As Long, ByRef ACAN As ByVal ADeviceHandle As Long, ByRef ACAN As TLIBCAN) As Long
Note: If you need the complete VB6.0 sample project source code, you can contact TOSUN application support at any time to get it.
3. Definition of the CAN/CANFD data structure
The CAN and CAN FD data structure is as follows:
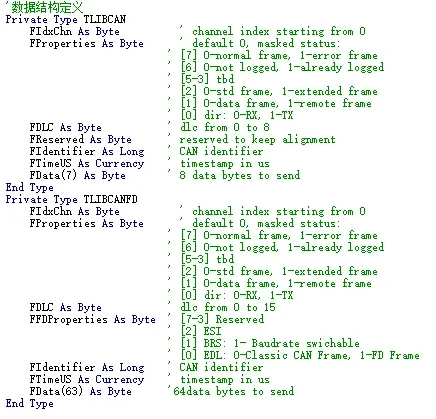
4. Call interface description
Description of the interfaces used in the demo:
initialize_lib_tscan_verbose
Function: Initialize the tscan library module. This function must be called to initialize the CAN module before other API functions can be called. This function is used in pair with finalize_lib_tscan.
Input Parameters:
AEnableFIFO[IN]: if or not enable FIFO mechanism, it is recommended to set it to True, otherwise users can not read the message through tsfifo_receive_xx function;
AEnableErrorFrame[IN]: whether to receive error frames. If set to False, the driver will discard the error frame directly;
AUseHWTime[IN]: whether to use the device connection time;
ATSType[IN] :Set it to False and it works;
Return value: None
finalize_lib_tscan
Purpose: To release the CAN module before exiting the program, paired with the create function initialize_lib_tscan.
Input parameters: none
Return value: None
tscan_get_device_info_detail
Role: Get device information
Input Parameters:
ADeviceIndex[IN]: device index value;
AManufacturer [OUT]: Manufacturer;
AProduct[OUT] : Product name;
ASerial[OUT] : Serial number;
ADeviceType[OUT] : Device type;
ADeviceName[OUT] : Device name;
ACANChannelCount[OUT] : Number of CAN channels;
AIsCANFD[OUT] : Whether CANFD is supported;
ALINChannelCount[OUT] : Number of LIN channels;
AFRChannelCount[OUT] : Number of FR channels;
AEthernetChannelCount[OUT] : Number of Ethernet channels;
tscan_connect
Function: Connects to a device and gets a unique handle to that device.
Input Parameters:
ADeviceSerial: get the device with the specified serial number, if the parameter is empty, it means to get any device in the connected state;
AHandle: device handle, subsequent operations on the hardware need to be based on this unique device handle;
tscan_scan_devices
Role: Scanning the number of devices online
Input Parameters:
ADeviceCount[OUT]: returns the number of online devices;
tscan_config_can_by_baudrate
Function: Configure the CAN bus baud rate
AHandle:Device handle;
AChnIdx:Application channel number;
ARateKbps:Baud rate;
A120OhmConnected: whether to enable the internal termination resistor;
tscan_transmit_can_sync
Function: Sends a CAN message and exits the function after detecting a successful send. This function returns success, which means that the CAN telegram must have been successfully sent to the CAN bus.
Input Parameters:
ADeviceHandle: device handle;
ACAN: The message data;
ATimeoutMS: synchronization wait timeout;
tscan_transmit_can_async
Function: Sends CAN messages asynchronously.
Input Parameters:
ACAN: CAN data packet. for TLIBCAN data composition, please check CAN, CANFD data composition section.
tscan_add_cyclic_msg_can
Function: Increase the number of messages sent in a cycle
Input Parameters:
ADeviceHandle: Handle to the operation device.
ACAN: CAN packet;
APeriodMS:Period value;
tscan_delete_cyclic_msg_can
Function: Deletes the periodic sending of CAN messages.
ACAN: Yes, periodic messages that need to be deleted;
tsfifo_receive_can_msgs
Function: Reads the message packets in the hardware cache.
Input Parameters:
ADeviceHandle: device handle;
ACANBuffers: Data Buffer, used to store the read messages, the Buffer needs to be created by the function caller;
ACANBufferSize: the size of the message buffer;
AChn:Target channel: for multi-channel devices, this function selects which channel to read the data, this parameter can be empty, the default is channel 1;
ARxTx:==0: only receive Rx messages; >0: Tx Rx messages are read back, this parameter can be empty, the default is to receive only Rx messages;
tsfifo_add_can_canfd_pass_filter
Function: Users need to call this function if they only want to receive messages with a specific ID.
Input Parameters:
ADeviceHandle: device handle;
AChnIdx: channel index;
AIdentifier: message identifier;
AIsStd: whether standard frame;
tsfifo_delete_can_canfd_pass_filter
Function: Cancel message filtering
Input Parameters:
ADeviceHandle: device handle;
AChnIdx: channel index;
AIdentifier: message identifier;
AIsStd: whether standard frame;
5.VB6.0 Demo realize effect
1) Click [Scan Devices], get the number of online devices and print it in the right control.
2) Click [Query Device Information] to get the specific information of the device and print it in the right control.
3) Click [Connect] to complete the hardware connection, print the connection success log and current device handle in the right control.
4) Click [Configure Hardware Channel] to complete the channel and baud rate settings.
5.) Click [Channel 1 Send Normal Message] to send 0x7B in a single frame.
6) Click [Receive CAN message from channel 2] to read the message data in the FIFO displayed in the ListBox below.
7) Click [Send Cycle Message] , and the message 0x29A is sent with a 50-ms cycle.
8) Click [Stop Cycle Transmission] ,message 0x29A stops cycle transmission.
(9) Click [Receive CAN message from channel 2], read the message data in the FIFO and display it in the ListBox below. As shown in Figure 3.
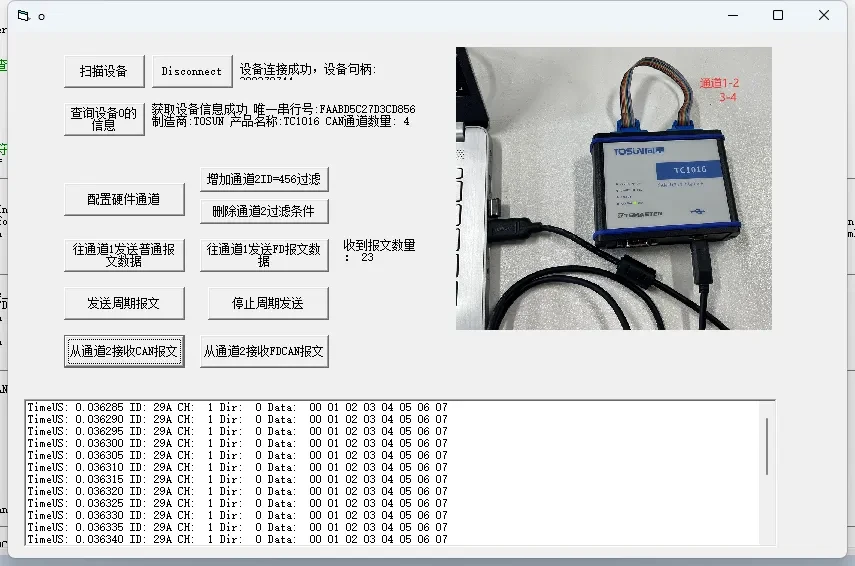
04. Troubleshooting (QA)
Q:After connecting, the device has been receiving messages, at this time, after I turn on filtering, then I go to send the message, when receiving the message, do I have to take out the data stored before until I read the filtered ID?
A: Right. Adding filtering actively clears out the old cache. Clear cache function: tsfifo_clear_can_receive_buffers.
Q: What does the return value of a function mean?
A:Function return value is 0 means the function execution is successful, other values means the function execution fails. Specific failure reason can be described according to the TSMaster system built-in constants to look up the table. If the return value is 3, it means "device not found", then you should check whether the current TSCAN device is connected normally.
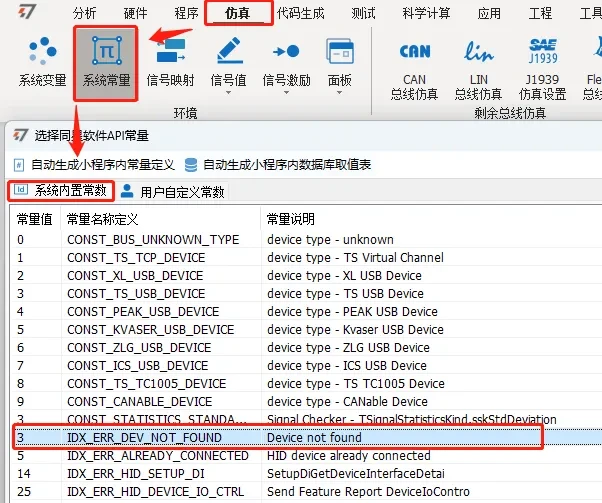